Hello Javascript
Javascript is an extremely powerful interpretted language that allows you to make your (mobile) web pages respond dynamically to user input.
Variable Types
- numbers - e.g. 777, 3.14
- Strings - e.g. 'This is a string'
- booleans - true, false
- objects
- null
- undefined
Javascript is a weakly typed language. Unlike java or c, you don't define the type when you declare a variable.
var x; // declaration
x = 1; // initialization
Number Literals
Arithmetic Operators
Javascript has standard arithmetic operators.
+
-
*
/
++
--
(see the Math object for additional math functions)
Comparison Operators
Javascript has standard comparison operators.
>
<
>=
<=
== // includes type-casting
!=
=== // does not include type-casting
!===
Logical Operators
Javascript has standard logical operators.
&& // logical AND
|| // logical OR
String Literals
var s;
s = 'How Are You';
s = s + ' Today';
s = s + ': ' + 2.71828183;
s.length; // string length
s.charAt(0); // => 'H', character at position, note starts with zero
s.substring(1,5); // => 'ow A'
s.indexOf('o'); // => 1, first occurence of 'o'
s.split('o'); // => ["H", "w Are Y", "u T", "day: 2.71828183"]
s.replace('Are', 'Be'); // => "How Be You Today: 2.71828183", Note this returns a new string
s // => "How Are You Today: 2.71828183"
s.toUpperCase() // => HOW ARE YOU TODAY: 2.71828183", Note this returns a new string
(use RegExp for additional functionality)
Arrays
var a; // BAD DECLARATION FOR AN ARRAY
var a = [];
var a = new Array();
a = [ 1, 20, 100, 7 ];
a[0]; // => 1
var b = a; // array assignment does not copy!!
b[0] = 'happy';
a; // => ['happy', 20, 100, 7], OOOOH, that can mess you up!
var b = a.slice(); // copies array a into a new array b
a.length; // => 4
a.reverse(); // => [7, 100, 20, "happy"]
a.sort(); // => [100, 20, 7, "happy"] , alphabetical!
Objects
Objects offer flexibility and portability to your code
var a = new Object();
var a = {};
var a= { x: 1, y: 2 };
a.x; // => 1
a = { x: 1, y: { z: 3} }; // object within an object
a.x; // => 1
a["x"]; // => 1
a.y; // => Object
a.y.z; // => 3
var b = a; // object assignment does not copy!
var b = Object.create(a);
var c = [a, 4, [ 5, 6] ]; // Objects in arrays
c[0]; = // => Object
var d = { x: 1, y: { m: 0, n: 2 }, z: [ 0, 5, 6]}; // Arrays in objects
d.z[1]; // => 5
Functions
Functions are how we do stuff
function square(x) {
return x*x;
};
square(4); // => 16
Functions are just another object and can be treated as variables
var s = square;
s(4); // => 16
Sometimes you'll see functions defined this way:
var square = function(x) {
return x*x;
};
Other times you'll see functions defined "in place"
setTimeout(function() {alert('time\'s up');}, 3000);
(also see the setInterval function)
Statements
Javascript also has the standard statements that you're used to from other languages.
While Loops
var x = 0;
while (x < 10) {
console.log('count=' + x);
x++;
};
(also see do/while loops)
For Loops
For loops are a shorthand while loop.
for (var x=0; x<10; x++) {
console.log('x=' + x);
};
For... In Statements
For/In statements are handy For loops for objects.
var obj = { name: 'sean', age: 34, title: 'professor', height: '5ft 10in'};
for (var x in obj) {
console.log(x + ': ' + obj[x]);
};
If / Else Statements
var x = 1;
if (x == 1) {
console.debug('x equals one');
} else {
console.error('x does not equal one');
};
Switch Statements
Switch statements can be used as shorthand if/else statements
switch(x)
{
case 1:
console.info('x==1');
break;
case 2:
console.warn('Warning: x==2');
break;
default:
console.error('Error: x is out of bounds');
};
Object Orientted Programming in Javascript
Object oriented programming permits encapsulation of variables and functions.
An object can be defined within it's constructor function.
function Rectangle( x1, y1, x2, y2 ) {
this.x1 = x1;
this.y1 = y1;
this.x2 = x2;
this.y2 = y2;
this.width = function() {
if (this.x2 > this.x1) {
return this.x2 - this.x1;
} else {
return this.x1 - this.x2;
}
};
};
var r = new Rectangle(0, 0, 1, 2); // => Rectangle
r.x1; // => 0
r.width(); // => 1
Javascript objects can also be defined with object prototpes. For more information, check out:
http://helephant.com/2009/01/18/javascript-object-prototype/
http://timkadlec.com/2008/01/using-prototypes-in-javascript/
Or search on google for javascript prototypes.
Document Object Model
So we've seen that Javascript is a powerful programming language for performing computations. What makes Javascript a powerful (mobile) web tool is its Document Object Model accessors. For lots of good examples, see http://www.w3schools.com/js/js_ex_dom.asp
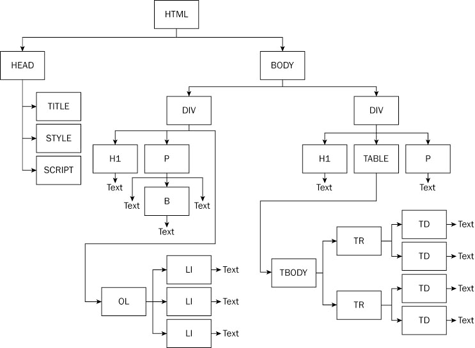
Lets start with a PhoneGap minimal example project and the following surrounding the innards of body:
<div id="main">
<h2 ...
</p>
</div>
Open it in your browser, open your firebug, and try the following in the console:
var main = document.getElementById("main");
main;
Now add a class-selector style to index.html
<style type="text/css">
.RedBackground
{
background:rgb(255,0,0); /*could also use background:#FF0000; or background:red;*/
}
</style>
Open it again in Firebug and type the following:
var main = document.getElementById("main");
main.className = "RedBackground";
Alternatively you can use this syntax to change class affiliations:
main.setAttribute("class", "");
main.setAttribute("class", "RedBackground");
main.getAttribute("class"); // => "RedBackground";
Now what if we want to get elements inside of our div?
mainkids = main.childNodes;
mainkids[0];
mainkids[1];
mainkids[0].textContent = "happy";
mainkids[1].style.display = "none";
mainkids[1].style.display = "inline";
mainkids[1].style.fontSize = "40pt";
mainkids[1].innerHTML = "<button>HI GUYS</button>"
You can also get elements by tag name and by class name:
document.getElementsByTagName("p");
document.getElementsByClassName("RedBackground");
Try out this example - DomExample
Be sure to view page source and watch the firebug console as you click on the page. Notice how the log changes when you resize the window and scroll.
Events
In addition to DOM manipulation, Javascript supports an event-driven interface.
There are many events that javascript supports, but we'll just cover some of the basics to get you started. For more events, see - http://www.w3schools.com/jsref/dom_obj_event.asp
onclick
The most common event triggers when you "click the mouse". On a mobile device this is when you tap the screen.
Lets start by creating a button. Add the following to your index.html
<button onclick="buttonClick();">Hoojadaddy?</button>
This html now has the onclick event registered to a function called "buttonClick". Now we need to make that function. Place the following within the <sript> tag of your HTML header.
function buttonClick() {
var answer = confirm("woot woot!");
if (answer){
alert("nice!");
}
else{
alert("whatevs...");
};
};
So now when you click the button, the buttonClick function is run and shows some alerts. Note that the onclick="" could have been set to call any function in your javascript code.
Cross-platform events
- onclick
- onmousedown
- onmouseup
Touch-platform events
(These may not work on your computer)
- touchstart
- touchend
- touchmove
Assigning a listener
document.body.addEventListener("click", function(){alert("body clicked");}, true);
Getting Window Dims / Position
document.documentElement.clientWidth; // width of the current browser window
pageYOffset; // This returns how far down the page the user has scrolled
Graphics
<canvas> element
http://www.w3schools.com/html5/html5_canvas.asp
http://billmill.org/static/canvastutorial/color.html
http://www.html5canvastutorials.com/
svg // scaled vector graphics
http://srufaculty.sru.edu/david.dailey/svg/intro/dynamic.html